My Coding >
Programming language >
Python >
Python graphics >
Wordcloud in Python
Wordcloud in Python
About WordCloud
wordcloud - Word cloud is a technique for visualising frequent words in a text where the size of the words represents their frequency.
To use wordcloud you need to install: - wordcloud - library to making word cloud
- matplotlib - library for plottong, used by other libraries, like wordcloud
I will also use the following libraries: - pillow - to create image
- wikipedia - to find a lot of text data
How to install WordCloud
Simple way to install wordcloud is to use pip install wordcloud or conda install -c conda-forge wordcloud, but for proper instalation of the last version is better to use the following commands
git clone https://github.com/amueller/word_cloud.git
cd word_cloud
pip install .
Draw simple wordcloud
import wikipedia
import matplotlib.pyplot as plt
from wordcloud import WordCloud, STOPWORDS
import re
wiki = wikipedia.page('Cebu') # Get text from wikipedia
text = wiki.content # Extract the plain text content of the page
text = re.sub(r'==.*?==+', '', text) # Clean text
# Define a function to plot word cloud
def plot_cloud(wordcloud):
plt.imshow(wordcloud) # Display image
# Generate word cloud
wordcloud = WordCloud( max_words=100, # number of words (200-default)
width = 1024, height = 800, # size of the image
random_state=1, # define colour selection
background_color='black', # background colour
colormap='rainbow', # type of world coloring
collocations=True, # use or not collocations of two words
stopwords = STOPWORDS # excluded words
).generate(text) # method if generating
plot_cloud(wordcloud) # draw image
wordcloud.to_file("cebu.jpg") # Save image
And the result is:
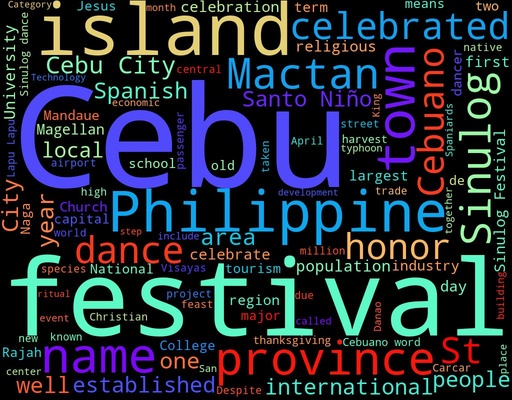 |
WordCloud for Cebu from Wikipedia Simple calculated WordCloud for text about Cebu from wikipedia.
Original image: 1024 x 800 |
Published: 2021-09-30 03:59:11 Updated: 2021-09-30 11:40:58
|