Converting polar field to cartesian coordinates
It is a common task to calculate vector fields in cartesian coordinates based on polar equations. For most visualisation and calculation procedures, cartesian coordinates are more natural and more straightforward, but for theoretical studies, polar (or cylindrical) coordinates often can be more helpful. In this example, I will show how to calculate vector fields in a cartesian coordinate system based on polar equations.
Equations of functions
First of all, let's make an example of polar functions:
\(F_r(r, \phi) = r^2 e^{-r^2}\)
\(F_\phi(r, \phi) = r^2 e^{-r^2}\sin(10\phi)\)
It is important to note, that in polar coordinates value of the function at R=0, should always be equal to 0 for radian and angular components, and can be any for vertical components (in cylindrical, 3D system).
Conversion
\(F_x = F_r \cdot \cos(\phi) - F_{\phi} \cdot \sin(\phi)\)
\(F_y = F_r \cdot \sin(\phi) + F_{\phi} \cdot \cos(\phi)\)
Coding
In Python, this task is pretty easy to solve with numpy.
Polar functions
For coding polar functions it is necessary to use numpy functions, rather than math functions, because we will apply them to a full domain in one go.
import numpy as np
import matplotlib.pyplot as plt
def Fr(r, phi):
f = r**2 * np.exp(-r**2)
return f
def Fphi(r, phi):
f = r**2 * np.exp(-r**2) * np.sin(10*phi)
return f
Cartesian domain
The final result will be stored in the Cartesian domain, so we need to create it. Personally, I prefer to use mgrid function from numpy. You can read what is the difference between mgrid and meshgrid. The domain will be squared from -3 to 3 with a grid size of 0.01
s, e, d, = -3, 3, 0.01
X, Y = np.mgrid[s:e:d, s:e:d]
Then we need to calculate values for \( \textbf{r}\) and \( \textbf{\phi}\) for every cell of the domain, and then calculate values of the functions for every given point.
r = np.sqrt(X**2 + Y**2)
phi = np.arctan2(Y, X)
Fr_values = Fr(r, phi)
Fphi_values = Fphi(r, phi)
After calculating all values in polar form, it is necessary to convert them to cartesian form according to the equation given. And also I will calculate the overall field value.
Fx = Fr_values * np.cos(phi) - Fphi_values * np.sin(phi)
Fy = Fr_values * np.sin(phi) + Fphi_values * np.cos(phi)
F = np.sqrt(Fx**2 + Fy**2)
Displaying
After calculation, we can visualise this field
plt.imshow(F, extent=(s,e,s,e), cmap='rainbow')
plt.title('F')
plt.show()
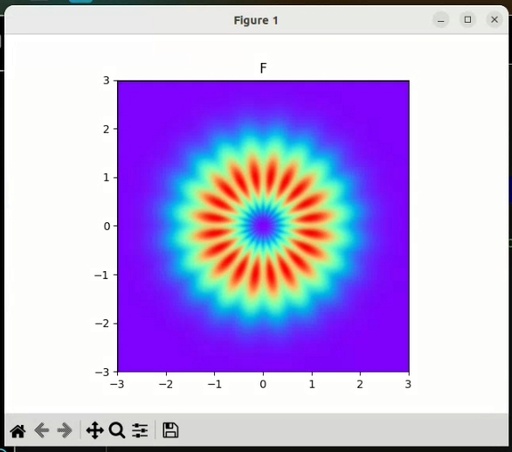
Example of polar field converted to cartesian system.
Original image: 586 x 517
Full code
This is a full working code:
import numpy as np
import matplotlib.pyplot as plt
def Fr(r, phi):
f = r**2 * np.exp(-r**2)
return f
def Fphi(r, phi):
f = r**2 * np.exp(-r**2) * np.sin(10*phi)
return f
s, e, d, = -3, 3, 0.01
X, Y = np.mgrid[s:e:d, s:e:d]
r = np.sqrt(X**2 + Y**2)
phi = np.arctan2(Y, X)
Fr_values = Fr(r, phi)
Fphi_values = Fphi(r, phi)
Fx = Fr_values * np.cos(phi) - Fphi_values * np.sin(phi)
Fy = Fr_values * np.sin(phi) + Fphi_values * np.cos(phi)
F = np.sqrt(Fx**2 + Fy**2)
plt.imshow(F, extent=(s,e,s,e), cmap='rainbow')
plt.title('F')
plt.show()
Published: 2023-09-14 02:37:30
Updated: 2023-09-14 02:51:36